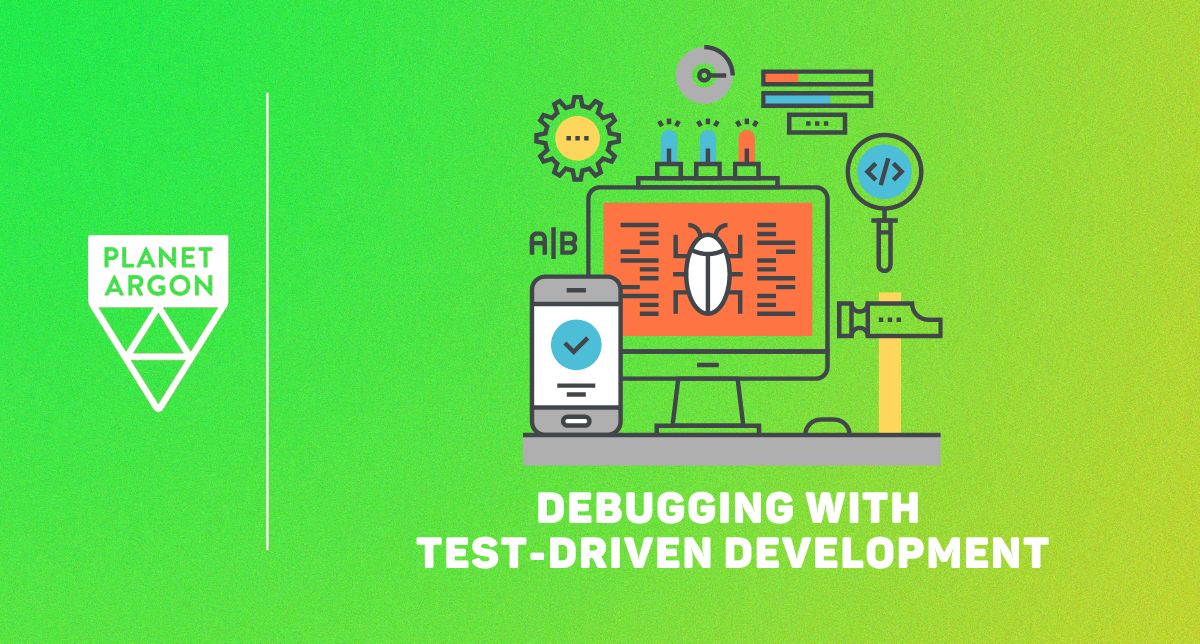
In the world of software development, bugs are an inevitable reality. They can cause frustration and delays, impacting a product's overall quality. However, by adopting a disciplined approach like Test-Driven Development (TDD), developers can effectively identify and fix bugs while maintaining the stability and reliability of their codebase. This article will explore some challenges you might encounter, steps for bug-fixing, and even some BONUS tips and tools.
TDD Basics
Test-Driven Development (TDD) is an approach where developers write automated tests before writing production code. It involves starting with a failing test case, then writing the minimum code required to pass the test. The code is subsequently refactored to enhance its structure and maintainability, ensuring all tests remain successful. While writing tests upfront requires additional time, it pays off in the long run by reducing time spent on debugging/reworking, thus improving productivity.
Debugging Challenges
Let's dig into some challenges you may encounter in debugging and how to overcome these challenges using TDD.
Feeling lost or overwhelmed when troubleshooting
Diving into a codebase without a lifeline can feel like diving head-first into a rolling ocean. It's easy to become overwhelmed. You may not know where to start making changes or how to track progress toward your goal.
Bugs seem to come up over and over
Sometimes you may solve an issue only to have it reappear again later. Having an automated test written for each issue ensures that issues you've fixed stay fixed.
Difficulty in understanding the basics of test-driven development (TDD)
If you're unfamiliar with TDD, you may have difficulty understanding how to utilize the process or what its benefits are. That means you may need to learn about test-driven development concepts such as writing test cases before production code, running failing tests to guide your development, and making code changes to make those tests pass.
Finding relevant resources
Many resources are available on debugging with TDD, but not all of them may be helpful or relevant. It can be challenging to find high-quality resources that are tailored to your specific needs and skill level.
Identifying the root cause of errors
Debugging can be challenging and time-consuming, especially if you're not experienced in troubleshooting code. If you struggle to identify the root cause of errors, it may take a significant amount of time to analyze the code and find the issue.
Difficulty in creating effective test cases
Writing effective test cases is an essential part of the TDD process. Still, writing test cases that cover all possible scenarios and catch all potential errors can also be challenging.
Wasting time on dead-ends
When writing code and debugging, you may find yourself pursuing many solutions that end up not working or have bugs or use cases not accounted for in the design. You may find yourself frantically making changes to your application and deploying them in the hopes that they fix your customers' issues without having any certainty that they will.
How I use TDD to Solve Bugs
The Test-Driven Development (TDD) process follows a cyclical approach. Here are 4 steps to follow when fixing a bug using TDD and real-world examples. Following them will give you a clear roadmap, guiding you towards efficient and permanent issue resolution while instilling a strong sense of direction throughout the process.
Step 1: Discover
The first step in bug-fixing with TDD is discovering the existence of a bug, which can be reported by a user, found during testing, or encountered during development. Once a bug is identified, it is crucial to thoroughly understand the issue. Developers should reproduce the bug consistently and gather as much information as possible, including error messages, logs, and the steps that led to the bug's occurrence. A clear and detailed understanding of the bug will guide the following steps and ensure a focused approach to resolving the issue.
Discover Example
In the example below, a bug is reported by the users of an application. A page doesn't load, and they see an error message.
To get more information on the error, the developer loads up the application in developer mode with the same data as the users and sees the following analysis:
Digging into the stack trace reveals that the error is occurring in a specific place in the code:
Some research reveals that this specific error occurs when using sort_by, and one of the data pieces it is being asked to sort by is Nil (blank). Now we have an idea of what is causing this error and can move on to writing a test.
Step 2: Test
With a clear understanding of the bug, the next step is to create a failing test case that reproduces the bug. This test case should be minimal, isolated, and focused solely on the specific issue at hand. Acting as an automated assertion, the test provides evidence of the bug's existence, serving as a safety net during the debugging process.
Creating the failing test case upfront helps prevent regression and ensures the bug is adequately fixed. When writing the test case, it is important to consider both the expected behavior and the potential edge cases associated with the bug. By covering all possible scenarios, developers can ensure comprehensive test coverage and increase the stability of their codebase.
Test Example
We find there is an existing test for this method:
The method is sorting on two data pieces. The test case does currently test any of the cases where either or both of those pieces are Nil. We add those cases in new context blocks, like so:
Now we run these tests. They fail with the same error that is occurring for the users:
This means our tests are successfully duplicating the same error that the users are experiencing. Now we can move on to making our code changes.
Step 3: Fix
With a failing test case in place, developers can now focus on fixing the bug. The key principle of TDD is to write the minimum amount of code necessary to make the test pass. Developers should focus solely on fixing the specific issue at hand, avoiding the temptation to implement additional features or make unrelated changes. By maintaining this laser-like focus, developers reduce the risk of introducing new bugs while ensuring the original issue is resolved efficiently.
Once the bug is fixed, developers should run the test suite to verify that the previously failing test case now passes. This step confirms that the bug has been successfully resolved and that the fix did not inadvertently introduce any new issues.
Fix Example
Adding sensible defaults to the sorting method allows it to continue in the case that it does not have the data it needs:
Re-running the test shows that all test cases now pass:
With our tests passing, we can move on to Quality Assurance.
Step 4: Quality Assurance
The final step in the bug-fixing process is to perform comprehensive quality assurance. QA involves thoroughly reviewing the entire codebase to ensure that the bug fix did not introduce any side effects or regressions. This includes running the entire test suite, performing integration tests, and conducting user acceptance testing if applicable.
Quality Assurance Example
In our developer server, we reload the page that had the error and see that it loads successfully now:
This error is now fixed! We run the application's entire test suite and deploy it to a staging server for further QA before showing it to the client for approval.
BONUS: Best Practices and Tools
Best Practices
- Keep the Red-Green-Refactor Cycle Short: In Test-Driven Development (TDD), aim to shorten the cycle of writing failing tests, making them pass, and refactoring the code. By focusing on small, incremental changes, developers can maintain a steady pace and avoid introducing unrelated changes or new bugs.
- Write Meaningful and Isolated Tests: Create meaningful and isolated test cases in TDD. Ensure tests clearly express intended behavior and have concise assertions. Isolated tests should not depend on the state or behavior of other tests, enabling independent execution and easier debugging.
- Refactor with Confidence: Rely on a comprehensive test suite to refactor code confidently in TDD. Tests serve as a safety net, detecting regressions or broken functionality. Regular refactoring improves code quality, reduces technical debt, and enhances maintainability.
Tools
- RSpec: RSpec is a testing framework for Ruby that offers a clean and expressive syntax for defining tests. It integrates seamlessly with Ruby on Rails, providing features like matchers and shared examples, enabling developers to write descriptive and readable test cases.
- Cypress: Cypress is a modern end-to-end testing framework for web applications. It offers an intuitive JavaScript API for writing tests that simulate user interactions and perform UI assertions. Cypress runs directly in the browser, facilitating real-time debugging and automatic reloading during test execution, making it a powerful tool for efficient end-to-end testing.
- Factory Bot: Factory Bot is a flexible library for creating and managing test data in Ruby. It simplifies the process of generating test objects with predefined attributes and associations. By offering a concise syntax for defining factories, Factory Bot allows developers to create consistent and realistic test data, enhancing the reliability and focus of their tests.