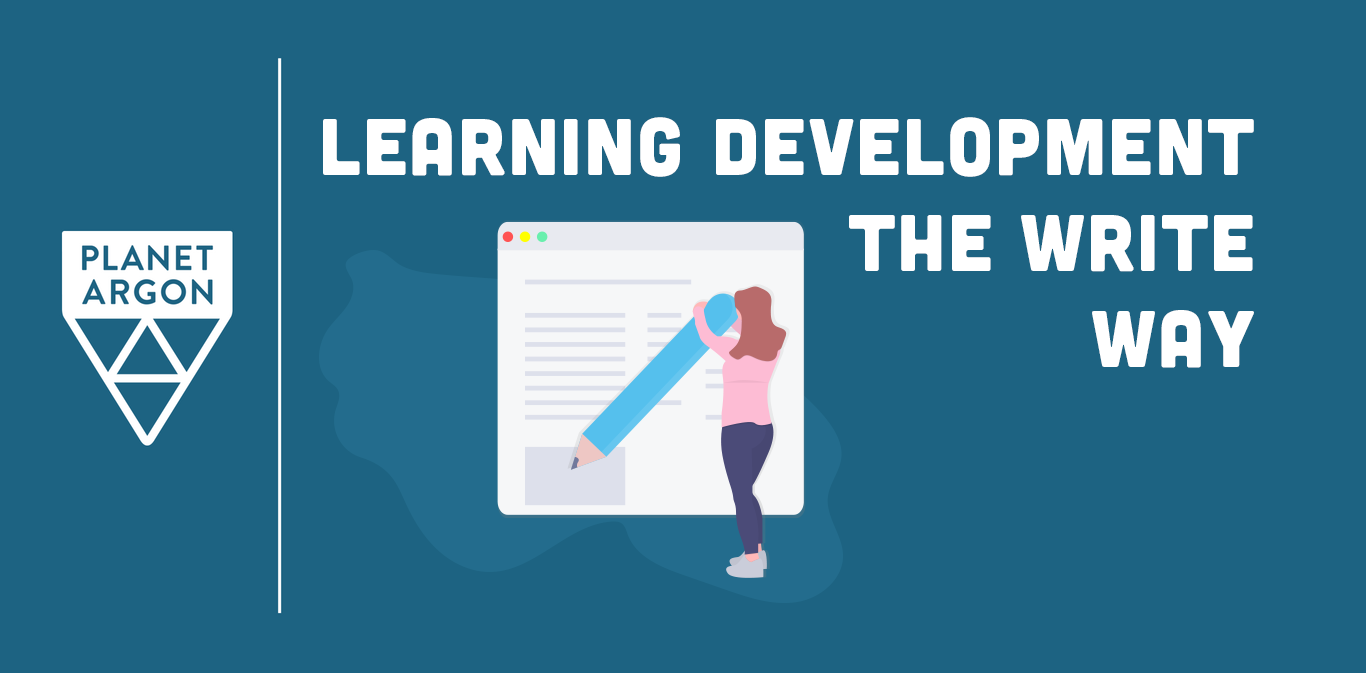
Retrain your brain
If there was an Oscars-like award ceremony for web developers, every winner’s speech would surely begin with, “I’d like to thank Google….”
Any hopeful programmer who’s spent more than a few minutes staring into the existential abyss of an inscrutable error message understands the siren-song of the internet search bar for troubleshooting.
Between StackOverflow, GitHub message boards, and a truly staggering volume of short-form tech blog posts (😀🤚) addressing every topic under the Silicon Valley sun, it’s a wonder our collective long-term memory brain lobes haven’t dried up and blown away like dusty, disused tumbleweeds.
Handy as these resources are, as a budding junior developer, I’ve found a good deal of growth has come from delaying that impulse to immediately copy/paste a problem into my browser and seek canned wisdom from the internet masses.
Don’t get me wrong. I’ve resolved my share of problems the tried and true Google way — often discovering clever solutions I never would have thought of on my own.
But, without taking some time to really wrestle with the problem first, a ready quick fix rarely ‘sticks’ in my brain, and I often discover I’ve fallen into the habit of relying more on Google than my own hard-won understanding of how something technical works.
As a growth exercise, then, each time I encounter the confusing, strange, or maddeningly broken, I try to approach it as an instructive puzzle to learn from rather than a problem task to check off a list.
Afterward, I try to also jot down a few notes describing the issue, how it was resolved, and what I learned in the process. This gives a reference anchor for later – a sort of mental bread crumb trail reminder of where I've been so far in my learning. It never feels like much at the time, but those notes add up and can serve as a handy reference guide to your ongoing journey as a developer.
In my personal experience, it’s these hard-won lessons in troubleshooting that settle the deepest in memory, ultimately furthering my understanding of how all this coding stuff fits together to make interesting, important tools for the world like Facebook, eBay, and AngryBirds.
So, in this post, I want to share several cool things I’ve learned about recently that were significant enough to warrant record keeping for later reference.
In particular, these represent points of insight that, once grasped, I feel furthered my general understanding of computing. You won’t find any quick fixes here, but hopefully, something sparks your curiosity and leads to an ‘aha!’ moment of your own you'd want to note for yourself or share with others.
Choose your $PATH wisely
The thing about computers is, they seem smart, but they’re not.
Not at all.
Your computer will do exactly as it's instructed. And then, only when you give specific, limited commands it can understand.
On modern machines, these commands are saved as tiny executable files and maintained in various directories scattered across the filesystem.
When you interact with a computer via the command line, it knows where to look for commands you specify by referencing a global system variable called $PATH
.
Test this out for yourself in the command line client of your choice:
$ echo $PATH
#my output:
>/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin:/opt/X11/bin
The output here looks weird. Like a confused web URL — a bunch of short strings separated by colons and slashes.
What’s going on here?
$PATH
is a shell variable that defines where your operating system looks for executable commands.
Simply put, it is a string of directory locations, separated by colons, that serve as the repositories for commands like echo
, mkdir
, touch
and others familiar to any command line user.
If a command isn’t found in the directories $PATH
knows about, the code won’t run:
#input
$ print-this-text "Hello!"
#output:
$ zsh: command not found: print-this-text
This error occurs because no executable file with the name 'print-this-text'
is found anywhere in the directories listed in $PATH
Why does this matter?
As a developer, you’ll almost certainly run into build errors on projects where the compiler is looking for a resource that it can’t find. Even after you’re sure you have the right dependencies installed, the computer (dummy that it is), will only look exactly where it’s told to — in $PATH
.
The solution? Update the value of $PATH
using the command line to include the missing directory:
#before:$ echo $PATH
> /usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin:/opt/X11/bin
#update command:
$ export PATH="/some/new/directory:$PATH"
#after:$ echo $PATH
> /some/new/directory:/usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin:/opt/X11/bin
Problem solved! Isn’t learning fun?
$PATH
is just one of many global variables used by an operating system to keep things running smoothly under the hood.
But, there’s one problem with the solution I showed you above. Shell variables updated through the command line always revert back to their original values when your session ends.
For the fix to stick, you’d have to enter that same command every time you need that directory included in $PATH
.
Wouldn’t you know it, there’s an easy way to make the change permanent, along with some other truly neat command line shortcuts.
This leads (rather conveniently) into my next discovery — Zsh!
Zsh is how we do it
The Z shell, or Zsh (pronounced ‘zeesh’), is a popular Unix shell command interpreter. It’s similar to the default Bash interpreter, but in addition to standard features, Zsh offers a long list of helpful developer tools and scripting shortcuts, including (courtesy of Wikipedia):
- Programmable command-line completion
- Sharing of command history among all running shells
- Spelling correction
- Various compatibility modes (Zsh can pretend to be a Bourne shell when run as
/bin/sh
) - Theme-able prompts, including the ability to put prompt information on the right side of the screen and have it auto-hide when typing a long command
- The built-in
where
command. Works like thewhich
command but shows all locations of the target command in the directories specified in$PATH
rather than only the one that will be used. - Named directories. This allows the user to set up shortcuts such as
~mydir
, which then behave the way~
and~user
do.
So what?
If this list doesn’t quite leave you feeling thrilled, let’s hone in on a single killer Zsh entry-point feature: the configuration file.
Once installed, Zsh places a hidden configuration file inside your user home directory. Every time you open a new shell instance, the interpreter runs through this file and executes any commands it finds and applies those to your session.
This has many exciting applications, but beginning with our small example, we’ll update $PATH
again just once (inside the configuration file) and have the change persist across all new shell sessions:
#open the Zsh config. file:
$ open ~/.zshrc
#in your text editor, paste the $PATH update command used earlier:
export PATH="/some/new/directory:$PATH"#save and close the file.
Congratulations, you magnificent coding genius… you’ve just written your first shell script!
Well, sort of. This is more of a command line shortcut than a full-fledged script. But take your victories wherever you can find them!
Let’s add a few more lines to the configuration file to save ourselves even more time on the command line:
#~/.zshrc contents:
export PATH="/some/new/directory:$PATH"
alias refresh='source ~/.zshrc'
alias make="mkdir"
alias detail='ls -a -l'
Taking advantage of the alias
variable assignment, commonly used commands can be saved to shorter or easier to remember placeholders that execute the same code when executed in the shell.
You can really go as nuts here as you want. There's no cost to having as few or as many shortcuts in your Zsh configuration file, so you can have fun playing around with your own setup. You'll be pleasantly surprised to learn how much faster your workflows become once you take some time to think through and optimize your command line processes.
Variable assignments can offer big time savers, but that alone barely scratches the surface of all that shell configuration has to offer. Zsh, in particular, has gained popularity due to its power and simplicity, as well as the huge variety of preconfigured themes made available by open source contributors.
Check out the popular Oh My Zsh! framework as a helpful entry point to get you started learning about what’s possible by seeing what other developers have created to increase their own shell productivity.
And if you’re truly looking to up your game as a master of the shell terminal, block out an hour or two of time and read through the excellent TutorialsPoint introduction to Unix.
Practice coming out of your shell
You don’t need to become a Unix expert to be a good programmer and web developer. In fact, if the topic feels too daunting or just plain boring to you, I’d recommend skipping it in favor of whatever else does tickle your fancy and helps get your projects running.
The point I’m making here is, whatever challenge you’re up against as you strengthen your developer skills, understanding the why is more helpful by far over the long term than copy/pasting the quick fix.
To borrow a popular Einstein quote:
If you can’t explain it simply, you don’t understand it well enough.
Personally, I remember things best and longest if I’m forced to uncomfortably wrestle with the unknown for a bit. Then, often after a Google deep-dive into the persisting problem, once broken things are working I’ll spend a few minutes free-writing a summary of the solution as I understand it.
These notes are just for me, so grammar and flow don’t matter as much as getting anything written for my future self to look at.
This is also a great way to develop the habit of general documentation, and plant idea seeds that could later become blog posts of your very own to help other developers. After all, someone has to write the how-to content we all (admitted or not) depend on — why not you?
Conclusion
All of us learn differently, but embracing the intimidating and unknown, learning from mistakes and roadblocks without ego, and documenting the process for yourself and the wider developer community are valuable skills worth prioritizing.
Remember — if being a developer were easy, it wouldn’t feel so hard.
Take some solace in the fact that none of this comes easily at first, and every small win is worthy of celebration.
Now, go build something cool!
Jared Reando is a spring intern at Planet Argon specializing in Rails/Ruby/React.js. You can learn more about Jared by connecting on LinkedIn or checking out his work on Github.