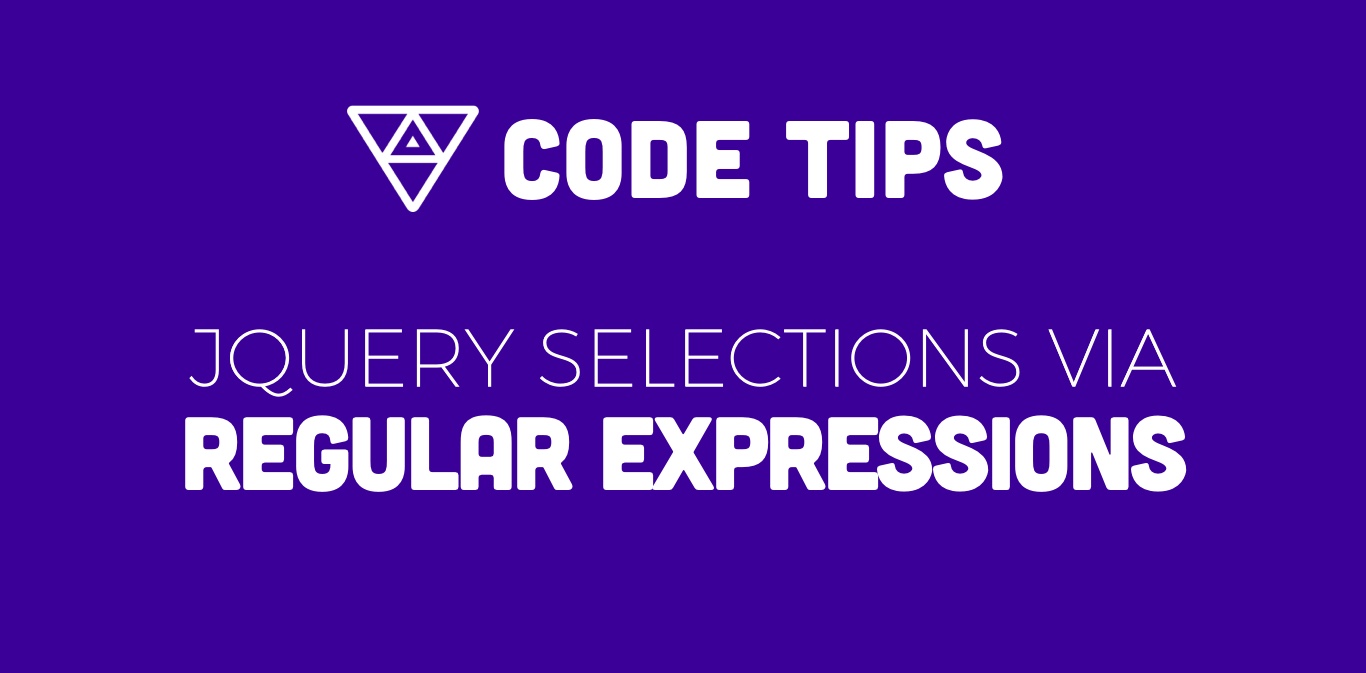
There are many convenient ways to target elements with jQuery selectors. Class and ID references are often suitable for the majority of use cases. Lets take a look at a simple alternative for something a bit more flexible.
JQuery's .filter() method is an often overlooked method that has proven handy when targeting elements whose selectors match a common pattern, as opposed to selecting them directly with a "." (class) or "#" (id) selector.
Recently we were looking for a way to target any images that we had uploaded to our site via the Square Space CMS. We identified a commonality across the qualifying elements. In this case they all had class names matching the following pattern 'featured_image', 'testimonial_image', etc. .
So we target the string '__image' with our regular expression.
// build our desired collection.
// matches both $('img.featured-testimonial__image') & $('img.featured-work__image')
const matches = $('img').filter(function() {
return this.className.match(/__image/);
});
// Do something to collection
$.each(matches, function(i, item) {
$item = $(item);
src = $item.attr('src');
$item.attr('src', src.replace('http://', '//'));
});
Just like that we have a script that will adapt to any image that follows the same pattern without any additional maintenance from us.